You might be trying to solve Redux problems while learning React. Kitze explains this better in the first minute of this video, where he gives some tips for those of us who are just starting in the world of React.
What is Redux?
Redux is a JavaScript library that allows you to manage the state of an application. We could also say that it's a data architecture pattern that allows state management. Although it's commonly associated with React, Redux is an agnostic JavaScript library, so it's very likely that you'll encounter Redux in Angular projects.
Why use Redux?
When we create applications in React, they are organized as a series of nested components, their nature is functional. In other words, they receive information through their arguments (props) and pass the information through their return values, and this is called: one-way binding, data is only transmitted from components to their children:
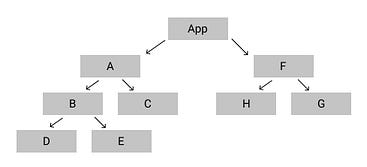
Let's look at an example of how React would behave with and without Redux.
React without Redux
So, let's imagine that in our component D we have an input that stores certain information in a state. This information will be available for the entire component D and its children, but what would happen if we need that information to also be present in component E?
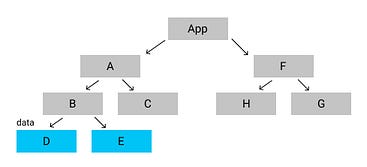
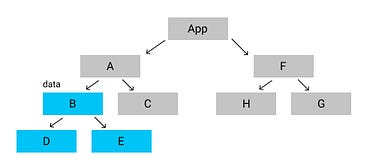
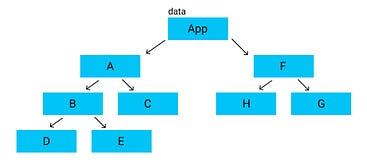
React with Redux
So, we need to have all that information in one place, so that it can be available in all our components, something like our single source of truth:
React Component tree with Redux
Having all that information in our Redux states, all our components will have access to the same information at all times.
Redux architecture pattern
Earlier we mentioned that Redux is an architecture pattern and we saw Redux as a blue box that stores states. Let's now see what we have inside our blue box and how it works:
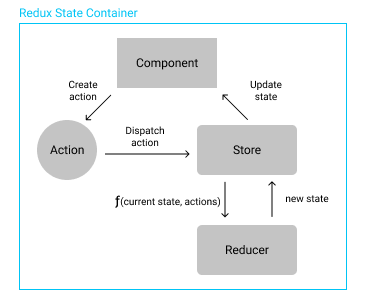
- We have a Component that will emit an Action, which can be called by some event: a click, for example.
- The Action goes to the Store where all the states are stored.
- The Store communicates to the Reducer the current state and which Action was executed.
- Then, the Reducer returns a new state modified by the action that was just executed.
- The state is updated in the Store.
- And the Store returns the new modified state to the component.
You might be facing a salad of new concepts, and it can be confusing, I tell you from experience. My recommendation is that you take the time necessary to understand what the Redux flow is, in a next post we will see the code behind our Redux architecture in a pet project.